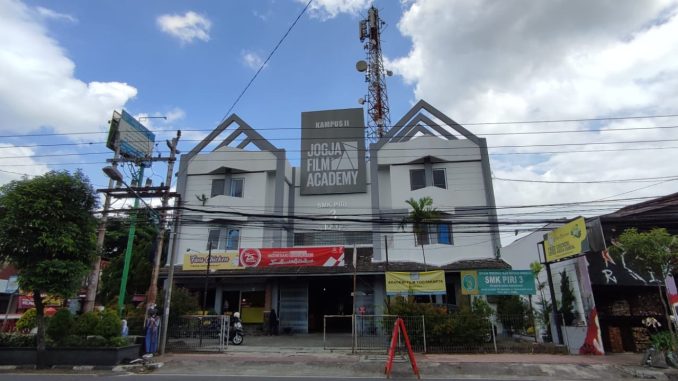
Bersama ini kami Universitasswasta.com menyampaikan informasi tentang Pendaftaran Kuliah Akademi Film Yogyakarta 2024/2025, sebagai berikut:
Akademi Film Yogyakarta
Akademi Film Yogyakarta (AFY) atau biasa disebut dengan Jogja Film Academy (JFA) lahir sebagai wujud tanggung jawab para professional kreator film untuk menyalurkan keilmuannya dengan melahirkan sineas-sineas baru. JFA mengajak para generasi baru bergabung dengan kami untuk menjawab tantangan di dunia film. Bertempat di kota dengan iklim pendidikan yang tinggi, JFA siap bersaing untuk melahirkan sineas-sineas masa mendatang. Kami adalah kampus film pertama di Yogyakarta.
Memiliki dosen yang profesional, bersemangat berbagi ilmu. JFA menghasilkan lulusan yang siap memperkaya wawasan masyarakat. Didirikan oleh para sineas Indonesia yang sudah diakui kehebatannya. Membangkitkan siswa untuk membuat film berdasarkan karakteristiknya masing-masing.
Pendaftaran Kuliah Akademi Film Yogyakarta TA 2024/2025
Syarat Pendaftaran
- Foto 4×6 background merah
- Foto 2R bebas sopan
- Biografi
- Scan Ijazah SMK/sederajat (asli)
- Foto 2R bebas sopan
- Scan KTP dan KK
Jadwal Pendaftaran
Program | Pendaftaran | Tes Akademik | Interview 1 | Pengumuman | Daftar Ulang |
Beasiswa | 5 Feb – 3 Mar 2024 | 6 Maret 2024 | 7 Maret 2024 | 8 Maret 2024 | 9 – 21 Maret 2024 |
Jalur Prestasi | 9 – 17 Maret 2024 | – | 21 Maret 2024 | 22 Maret 2024 | 23 Maret – 4 April 2024 |
Gelombang Khusus | 18 – 31 Maret 2024 | 3 April 2024 | 4 April 2024 | 5 April 2024 | 6 – 25 April 2024 |
Gelombang 1 Sesi 1 | 1 – 21 April 2024 | 24 April 2024 | 25 April 2024 | 26 April 2024 | 27 April -9 Mei 2024 |
Gelombang 1 Sesi 2 | 22 April – 5 Mei 2024 | 7 Mei 2024 | 8 Mei 2024 | 10 Mei 2024 | 11 – 19 Mei 2024 |
Gelombang 1 Sesi 3 | 6 – 19 Mei 2024 | 21 Mei 2024 | 22 Mei 2024 | 24 Mei 2024 | 25 Mei – 6 Juni 2024 |
Gelombang 2 Sesi 1 | 20 Mei – 2 Juni 2024 | 5 Juni 2024 | 6 Juni 2024 | 7 Juni 2024 | 8 – 20 Juni 2024 |
Gelombang 2 Sesi 2 | 3 – 16 Juni 2024 | 19 Juni 2024 | 20 Juni 2024 | 21 Juni 2024 | 22 Juni – 4 Juli 2024 |
Gelombang 2 Sesi 3 | 17 – 30 Juni 2024 | 3 Juli 2024 | 4 Juli 2024 | 5 Juli 2024 | 6 Juli – 18 Juli 2024 |
Gelombang 3 Sesi 1 | 1 – 14 Juli 2024 | 17 Juli 2024 | 18 Juli 2024 | 19 Juli 2024 | 20 Juli – 1 Agustus 2024 |
Gelombang 3 Sesi 2 | 15 – 28 Juli 2024 | 31 Juli 2024 | 1 Agustus 2024 | 2 Agustus 2024 | 3 – 22 Agustus 2024 |
Gelombang 3 Sesi 3 | 29 Juli – 11 Agust 2024 | 14 Agustus 2024 | 15 Agustus 2024 | 16 Agustus 2024 | 17 – 23 Agustus 2024 |